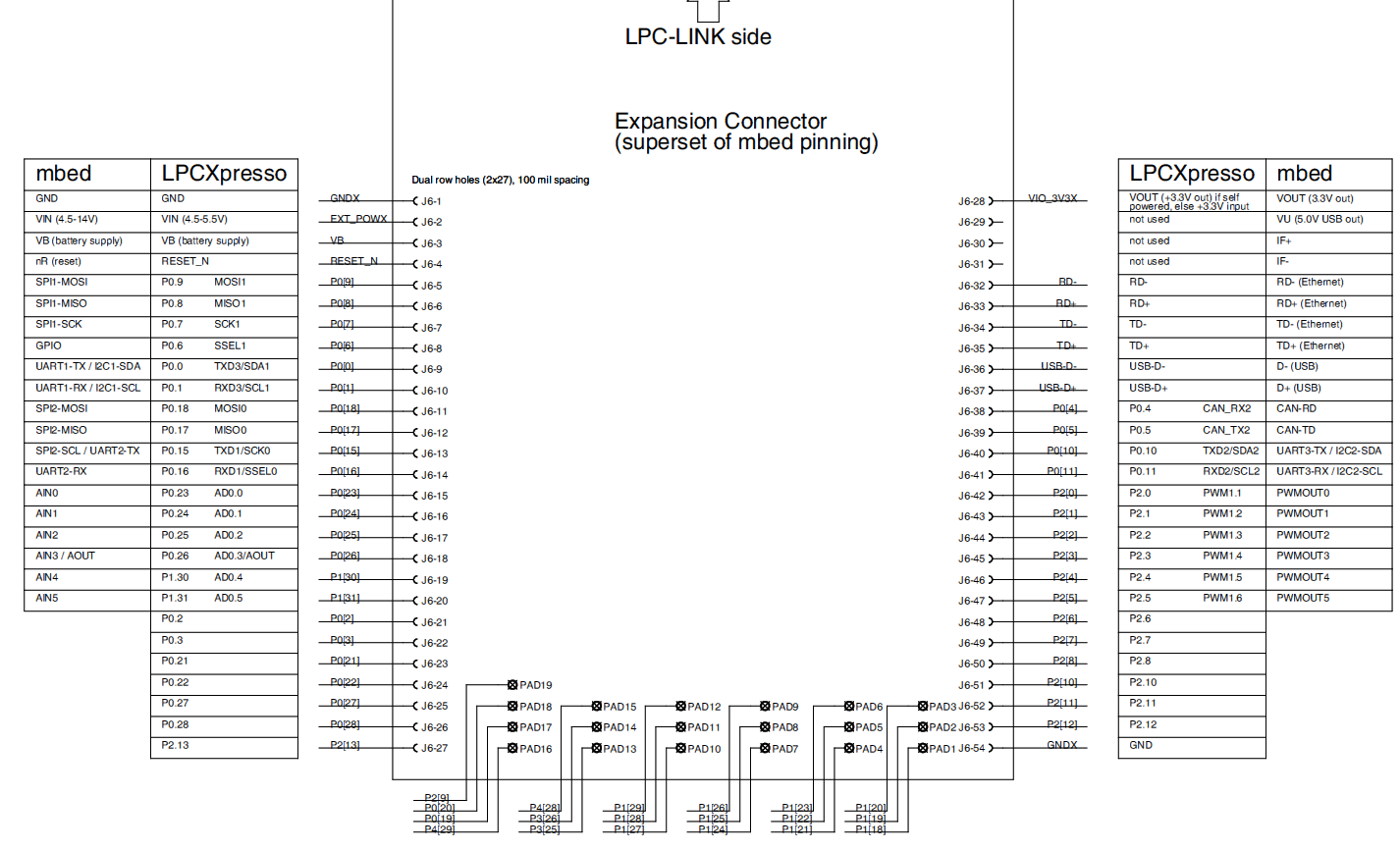
Aqui se ve como esta distribuido los pines en el LPC 1769 y sus respectivos nombres.
///////////////////CODIGO PARA TECLADO MATRICIAL 4X4 en LPC1769 /////////////
/* Standard --diese code war am 12.03.2014 entworfen-- includes.
* Codigo hecho por Jorge Morales 12 Marzo 2014 */
* wenn du Zweifel hast, schreib mir jorge_morales92@hotmail.com*/
#include <string.h>
#ifdef __USE_CMSIS
#include "LPC17xx.h"
#endif
/* FreeRTOS includes. */
#include "FreeRTOS.h"
#include "task.h"
#include <cr_section_macros.h>
#include <NXP/crp.h>
/* Example includes. */
#include "GPIO-output-and-software-timers.h"
#include "UART-interrupt-driven-command-console.h"
#include "UART-operation-modes-test.h"
#include "SPI-writes-to-7-seg-display.h"
#include "I2C-coordinator.h"
#include "FreeRTOS_CLI.h"
/* Library includes. */
#include"lpc17xx_gpio.h"
#include"lpc17xx_timer.h"
#include"lpc17xx_timer.h"
#include "display.h"
#include "lpc17xx_adc.h"
#include "lpc17xx_pinsel.h"
static uint32_t ulClocksPer10thOfAMilliSecond = 0UL;
// declare prototipos de funciones
void CONFIG_GPIO (void);
void rastreo(void *pvParameters );
void scan(void *pvParameters );
#define mainDELAY_LOOP_COUNT (0xfffff)
#define Entrada 0
#define Salida 1
// PINES DE PUERTO 0 COMO ENTRADA
#define PIN0_8 (1 << 8)
#define PIN0_9 (1 << 9)
#define PIN0_10 (1 << 10)
#define PIN0_11 (1 << 11)
//PINES DE PUERTO 2 COMO SALIDA
#define PIN2_8 (1 << 8)
#define PIN2_10 (1 << 10)
#define PIN2_11 (1 << 11)
#define PIN2_12 (1 << 12)
// DEFINICION DE PUERTOS
#define PUERTO_0 ((unsigned int)0)
#define PUERTO_2 ((unsigned int)2)
// definicion de variables a usar
int fila;
int tecla;
// variables para guardar el valor del puerto 0
uint32_t a;
uint32_t b;
uint32_t c;
uint32_t d;
//definicion de valores a usar en el Display
uint32_t cero = 0x3F;
uint32_t uno = 0x06;
uint32_t dos = 0x5B;
uint32_t tres = 0x4F;
uint32_t cuatro = 0x66;
uint32_t cinco = 0x6D;
uint32_t seis = 0x7D;
uint32_t siete = 0x07;
uint32_t ocho = 0x7F;
uint32_t nueve = 0x67;
xTaskHandle xTask2Handle;
xTaskHandle xHandleScan;
int main( void )
{
CONFIG_GPIO();
xTaskCreate(scan, "scan", 240, NULL, 1, &xHandleScan); //&xHandleScan funciona como una etiqueta para poder manejar la tarea
xTaskCreate(rastreo, "rastreo", 240, NULL, 2, &xTask2Handle);
vTaskStartScheduler();
for( ;; );
return 0;
}
/*------Hecho por Jorge Morales 11 Marzo 2014----------------------------------------------------*/
void CONFIG_GPIO (void)
{
PINSEL_CFG_Type PinCfg;
// PUERTO 0.8 COMO ENTRADA
PinCfg.Portnum = 0;
PinCfg.Pinnum = 8;
PinCfg.Pinmode = 0;
PinCfg.Funcnum = 0;
PinCfg.OpenDrain = PINSEL_PINMODE_NORMAL;
PINSEL_ConfigPin(&PinCfg);
// PUERTO 0.9 COMO ENTRADA
PinCfg.Portnum = 0;
PinCfg.Pinnum = 9;
PinCfg.Pinmode = 0;
PinCfg.Funcnum = 0;
PinCfg.OpenDrain = PINSEL_PINMODE_NORMAL;
PINSEL_ConfigPin(&PinCfg);
// PUERTO 0.10 COMO ENTRADA
PinCfg.Portnum = 0;
PinCfg.Pinnum = 10;
PinCfg.Pinmode = 0;
PinCfg.Funcnum = 0;
PinCfg.OpenDrain = PINSEL_PINMODE_NORMAL;
PINSEL_ConfigPin(&PinCfg);
// PUERTO 0.11 COMO ENTRADA
PinCfg.Portnum = 0;
PinCfg.Pinnum = 11;
PinCfg.Pinmode = 0;
PinCfg.Funcnum = 0;
PinCfg.OpenDrain = PINSEL_PINMODE_NORMAL;
PINSEL_ConfigPin(&PinCfg);
////////////////////////////////////////////////////////////////
// PUERTO 2.8 COMO SALIDA
PinCfg.Portnum = PINSEL_PORT_2;
PinCfg.Pinnum = PINSEL_PIN_8;
PinCfg.Pinmode = PINSEL_PINMODE_TRISTATE;
PinCfg.Funcnum = PINSEL_FUNC_0;
PinCfg.OpenDrain = PINSEL_PINMODE_NORMAL;
PINSEL_ConfigPin(&PinCfg);
// PUERTO 2.10 COMO SALIDA
PinCfg.Portnum = PINSEL_PORT_2;
PinCfg.Pinnum = PINSEL_PIN_10;
PinCfg.Pinmode = PINSEL_PINMODE_TRISTATE;
PinCfg.Funcnum = PINSEL_FUNC_0;
PinCfg.OpenDrain = PINSEL_PINMODE_NORMAL;
PINSEL_ConfigPin(&PinCfg);
// PUERTO 2.11 COMO SALIDA
PinCfg.Portnum = PINSEL_PORT_2;
PinCfg.Pinnum = PINSEL_PIN_11;
PinCfg.Pinmode = PINSEL_PINMODE_TRISTATE;
PinCfg.Funcnum = PINSEL_FUNC_0;
PinCfg.OpenDrain = PINSEL_PINMODE_NORMAL;
PINSEL_ConfigPin(&PinCfg);
// PUERTO 2.12 COMO SALIDA
PinCfg.Portnum = PINSEL_PORT_2;
PinCfg.Pinnum = PINSEL_PIN_12;
PinCfg.Pinmode = PINSEL_PINMODE_TRISTATE;
PinCfg.Funcnum = PINSEL_FUNC_0;
PinCfg.OpenDrain = PINSEL_PINMODE_NORMAL;
PINSEL_ConfigPin(&PinCfg);
PinCfg.Portnum = PINSEL_PORT_1;
PinCfg.Pinnum = PINSEL_PIN_31;
PinCfg.Pinmode = PINSEL_PINMODE_TRISTATE;
PinCfg.Funcnum = PINSEL_FUNC_0;
PinCfg.OpenDrain = PINSEL_PINMODE_NORMAL;
PINSEL_ConfigPin(&PinCfg);
//como salida para teclado E puerto 2.8 2.10 2.11 2.12
GPIO_SetDir(PUERTO_0, PIN0_8 , Entrada); // (0, 1<<8, 0);
GPIO_SetDir(PUERTO_0, PIN0_9 , Entrada);
GPIO_SetDir(PUERTO_0, PIN0_10 , Entrada);
GPIO_SetDir(PUERTO_0, PIN0_11 , Entrada);
//como salidas puertos --2.8 --2.10 --2.11 --2.12
GPIO_SetDir(PUERTO_2, PIN2_8 , Salida);
GPIO_SetDir(PUERTO_2, PIN2_10 , Salida);
GPIO_SetDir(PUERTO_2, PIN2_11 , Salida);
GPIO_SetDir(PUERTO_2, PIN2_12 , Salida);
GPIO_SetDir(1, 1<<31 , Salida);
// configuracion para display como entradas y salidas
/*GPIO_SetDir(0,0x000007F,1);
GPIO_ClearValue(0, 0x7F);*/
GPIO_SetDir(2,0x000007F,1);
GPIO_ClearValue(2, 0x7F);
GPIO_SetDir(1,0xF0000000,1);
GPIO_ClearValue(1, 0xF0000000);
}
void rastreo( void *pvParameters )
{
int i;
for(;;)
{
for(i=1; i<5;i++)
{
GPIO_ClearValue(0, 0xF7F);
GPIO_ClearValue(2, 0x1E00);
switch (i)
{
case 1: GPIO_SetValue(PUERTO_2, 0x1E00); fila=1; break;
case 2: GPIO_SetValue(PUERTO_2, 0x1B00); fila=2; break;
case 3: GPIO_SetValue(PUERTO_2, 0x1700); fila=3; break;
case 4: GPIO_SetValue(PUERTO_2, 0x0F00); fila=4; break;
default: break;
}
//GPIO_ClearValue(1, 0x80000000);
vTaskSuspend(xTask2Handle);
}
}
}
void scan( void *pvParameters )
{
volatile unsigned long ul;
for(;;)
{
a=GPIO_ReadValue(PUERTO_0)&(1<<11);//columna 4 P0.11
b=GPIO_ReadValue(PUERTO_0)&(1<<10);//columna 3 P0.10
c=GPIO_ReadValue(PUERTO_0)&(1<<9); //columna 2 P0.9
d=GPIO_ReadValue(PUERTO_0)&(1<<8); //columna 1 P0.8
//Columna 4 valores 1 4 7 F
if (a==0)
{
switch (fila)
{
case 0: break; //TECLA error!
case 1: displayNumber(1); tecla=1; break; //TECLA 1
case 2: displayNumber(4); tecla=4; break; //TECLA 4
case 3: displayNumber(7); tecla=7; break; //TECLA 7
case 4: displayNumber(15); tecla=15; break; //TECLA F
default: break;
}
}
//Columna 3 valores 2 5 8 0
if(b==0)
{
switch (fila)
{
case 0: break; //TECLA error!
case 1: displayNumber(2); tecla=2; break; //TECLA 2
case 2: displayNumber(5); tecla=5; break; //TECLA 5
case 3: displayNumber(8); tecla=8; break;; //TECLA 8
case 4: displayNumber(0); tecla=0; break; //TECLA 0
}
}
/*------Hecho por Jorge Morales 11 Marzo 2014----------------------------------------------------*/
//Columna 2 valores 3 6 9 E
if(c==0)
{
switch (fila)
{
case 1: displayNumber(3); tecla=3; break; //TECLA 3
case 2: displayNumber(6); tecla=6; break; //TECLA 6
case 3: displayNumber(9); tecla=9; break; //TECLA 9
case 4: displayNumber(14); tecla=14; break; //TECLA E 0x79 0b01111001
}
}
//Columna 1 valores A B C D
if(d==0)
{
switch (fila)
{
case 1: displayNumber(10); tecla=10; break; //TECLA A 0x77
case 2: displayNumber(11); tecla=11; break; //TECLA B 0x7C
case 3: displayNumber(12); tecla=12; break; //TECLA C 0x39 0b00111001
case 4: displayNumber(13); tecla=13; break; //TECLA D 0x5E 0b01011110
}
}
/*------Hecho por Jorge Morales 1455666 11 Marzo 2014--------------------------------*/
vTaskResume(xTask2Handle);
}
}
/*********************************************************/
//************************************
///////////////////////////////////////////////////////////////////////////////
Libreria Display.h y Display.c
CREAN UNA LIBRERIA LLAMADA DISPLAY.H y la dejan en blanco
DESPUES CREAN UN ARCHIVO EN .C Y LE COLOCAN EL CODIGO DE ABAJO
//////////////////////////////////////////////////////////////////////////////
#include "display.h"
int displayNumber(int num)
{
int cero = 0x3F;
int uno = 0x06;
int dos = 0x5B;
int tres = 0x4F;
int cuatro = 0x66;
int cinco = 0x6D;
int seis = 0x7D;
int siete = 0x07;
int ocho = 0x7F;
int nueve = 0x67;
int A= 0x77;
int B= 0x7C;
int C= 0x39;
int D= 0x5E;
int E= 0x79;
int F= 0x71;
GPIO_ClearValue(2, 0x7F);
switch (num) {
case 1: GPIO_SetValue(2, uno); break;
case 2: GPIO_SetValue(2, dos); break;
case 3: GPIO_SetValue(2, tres); break;
case 4: GPIO_SetValue(2, cuatro); break;
case 5: GPIO_SetValue(2, cinco); break;
case 6: GPIO_SetValue(2, seis); break;
case 7: GPIO_SetValue(2, siete); break;
case 8: GPIO_SetValue(2, ocho); break;
case 9: GPIO_SetValue(2, nueve); break;
case 0: GPIO_SetValue(2, cero); break;
case 10: GPIO_SetValue(2, A); break;
case 11: GPIO_SetValue(2, B); break;
case 12: GPIO_SetValue(2, C); break;
case 13: GPIO_SetValue(2, D); break;
case 14: GPIO_SetValue(2, E); break;
case 15: GPIO_SetValue(2, F); break;
}
}
////Si tienes dudas de como conectar solo observa el codigo ahi dice que pines se conectan y observa a imagen de distribucion de pines ////////
No hay comentarios.:
Publicar un comentario